|
What exactly are we trying to do here?
As we
covered in the first part of The Most Simple
Intro to CGI, in order to do a CGI based application, such
as a fill in the blanks HTML form, we must write a special
type of program. Since what we are going to be creating is
rather simple, we can also refer to it as a script. I
am sure you now feel better knowing you will be
scripting instead of programming.
Like it or not, you are going to have to learn just a
little bit about programming. I will try to make it as simple
as possible. I will explain what each part of our little
project does, but not in any great depth. Trust me- you would
die of boredom if I did not spare you the details.
The language our script is going to use is called PERL.
PERL is an ideal language for writing CGI scripts. You can
also write CGI scripts in other languages such as C, or even
the unix shell.
So here are our objectives for this section:
- Discuss what we need to make our script work.
- Create a simple form to collect our data.
- Write a script that processes this information and
returns it to the browser as an HTML page.
What do we need to make our script work?
The project
we will be doing has been designed for a Unix based server. It
will work just fine with Linux, SCO, etc... There are a few
questions that you must seek out the answers to before we can
get started.
- What is your main URL for cgi? It should be something
like http://www.yourdomain.com/cgi-bin/. If not, then
you will need to find out from your administrator what it
is, and make the minor corrections to the script where
needed.
- What is the UNIX PATH to your cgi-bin directory. You
need to know where to either cd or FTP to, to
do your work. For me- it is
/usr/dom/bignose/cgi-bin/.
- Do you have the language PERL on your system. You
will also need to know what directory it is in. To find out
quickly, issue the command
type perl The result on my system
is /usr/local/bin/perl. This is where it is on most
systems. If it is different, Make a note of it so you can
change the first line of our script.
- Do your scripts need a certain extension? In
otherwords, must the script end with .cgi?
- IMPORTANT: If you are using FTP to transfer the
program from your PC to a UNIX server, make sure you use
ASCII mode and NOT BINARY! DOS uses an extra character
(CR/LF) to indicate end of line, where UNIX only uses (LF).
Using ASCII removes the extra character. If this is not
done, your script most likely will fail to run.
Okay, we now have all of the techie systems stuff
out of the way and we can get on with dealing with the meat of
our project.
Creating an input form with HTML
The first
part of our actual project is to create a simple form. This
will allow you to call the script for testing purposes. Please
be very careful not to change anything in the form. The source
code is right under the graphic below. To make your life easy,
just cut and paste. Place the HTML file in the directory where
you normally place your web pages.
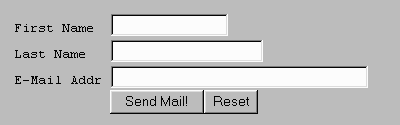 Not a real form- just an amazing
simulation!
Source Code for myscript.html
|
<HTML>
<BODY>
<FORM METHOD="POST" ACTION="/cgi-bin/myscript.cgi">
<PRE>
First Name <INPUT TYPE="text" NAME="fname" MAXLENGTH=15 SIZE=15>
Last Name <INPUT TYPE="text" NAME="lname" MAXLENGTH=20 SIZE=20>
E-Mail Addr <INPUT TYPE="text" NAME="email" MAXLENGTH=35 SIZE=35>
<INPUT TYPE="submit" VALUE="Send Mail!">
<INPUT TYPE="reset" value=" Clear-Form">
</PRE>
</FORM>
</BODY>
</HTML>
NOTE: The only change you will have to make is if you can't
refer to your script as /cgi-bin/myscript.cgi It may have
to be http://www.yourdomain.com/cgi-bin/myscript.cgi,
or whatever the answer was to, "what is your URL for cgi?"
A brief tangent...If you look at the
source code above you will notice that METHOD=POST. You may
in looking at other programs see METHOD=GET. I strongly urge
you to use the METHOD=POST. The main difference is how the
information is sent to the cgi script. GET places the
information in an environmental variable, like PATH
or HOME. Some unix systems limit the length of these
variables to 80 or 255 characters, or whatever length. If
your information is too long to fit- your script will not
work! The POST method sends the information as a stream-
like a school of fish. This means there is no
practical length limit. There is a limit that is set
by the administrator, but it is usually VERY big.
|
As we
mentioned in the last installment, the whole purpose of the
form is to feed data to our highly intelligent killer whale,
KJ. If you examine the source code above, and look at the
illustration below, we have a fish for each piece of
information must be fed to KJ. I want you to think of the
three fish as being a school.
If we look at
our school of fish, we can see that there are three. Their
names are fname, lname, and email. The information they carry
is in their bellies. If you were to look at what this school
of fish actually looked like, it would be something like this:
fname=bruce&lname=gronich&email=bnb@xyz.com
This is how the browser transmits your form's
information to our cgi script. If there were a comments field,
this stream could be much longer.
Dealing with our form's data This
brings us to the gory part of our project- the actual script
itself. The first section we will look at is the part that
decodes- or guts the incoming fish. I warned you. I have been
using this tidbit for so long I don't remember where I found
it.
In this first part of our script, we must take the incoming
school of fish, and let KJ know where one fish starts and
another one ends. The & signs are called delimiters
or field separators. Not only that, but we also have to find
out the name of each fish and what it holds in its belly.
The = (equal) signs mean that a value belongs to a
particular field, such as fname being equal to bruce.
It is beyond the scope of this introduction to teach you
the PERL language, but I do encourage you to learn more about
it when you have a chance.
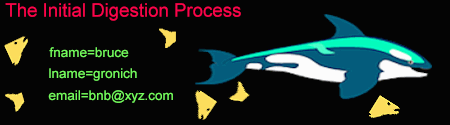
Source Code Part 1 of myscript.cgi
|
***NOTE: Be sure that your "perl" is in the right place- or change
the first line of the script.
IT IS IMPORTANT that the line that has perl's location
BE THE VERY FIRST LINE in the script!!!!
#!/usr/local/bin/perl
read(STDIN,$temp,$ENV{'CONTENT_LENGTH'});
@pairs=split(/&/,$temp);
foreach $item(@pairs)
{
($key,$content)=split(/=/,$item,2);
$content=~tr/+/ /;
$content=~s/%(..)/pack("c",hex($1))/ge;
$fields{$key}=$content;
}
|
Now that our
fish have been divided up into nice identifiable and
digestible pieces, what's next?
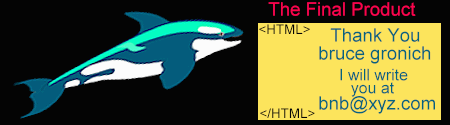
Our next and final objective is to add the part of the
program that prints out the HTML document that will be
returned to the browser.
Please look very closely at the code, it may help you make
sense of the first part of the program.
Source Code Part 2 of myscript.cgi
print "Content-type: text/html\n\n";
print "<HTML>\n";
print "<BODY BGCOLOR=#FFFFFF>\n";
print "<CENTER>\n";
print "THANK YOU<BR>\n";
print "$fields{fname} $fields{lname}</BR>";
print "I will write<BR>\n";
print "you at<BR>\n";
print "$fields{email}<BR>\n";
print "</CENTER>\n";
print "</BODY></HTML>";
Now, add this
source code into your copy of myscript.cgi and you will
be all set to try it out! Be sure to issue the command to
properly set the permissions.
chmod 755 myscript.cgi
IMPORTANT: If you are using FTP to transfer the
program from your PC to a UNIX server, make sure you use
ASCII mode and NOT BINARY! DOS uses an extra character
(CR/LF) to indicate end of line, where UNIX only uses (LF).
Using ASCII removes the extra character. If this is not
done, your script most likely will fail to run.
If you experience any errors, the odds are you are missing
some punctuation mark. Check your work carefully.
- Bruce
The Entire Source Code to myscript.cgi
#!/usr/local/bin/perl
read(STDIN,$temp,$ENV{'CONTENT_LENGTH'});
@pairs=split(/&/,$temp);
foreach $item(@pairs)
{
($key,$content)=split(/=/,$item,2);
$content=~tr/+/ /;
$content=~s/%(..)/pack("c",hex($1))/ge;
$fields{$key}=$content;
}
print "Content-type: text/html\n\n";
print "<HTML>\n";
print "<BODY BGCOLOR=#FFFFFF>\n";
print "<CENTER>\n";
print "THANK YOU<BR>\n";
print "$fields{fname} $fields{lname}</BR>";
print "I will write<BR>\n";
print "you at<BR>\n";
print "$fields{email}<BR>\n";
print "</CENTER>\n";
print "</BODY></HTML>";
|
|
|