The "BallWorld" class - version 1 We shall design out BallWorld class version 1 to have the following variables and methods: class BallWorld | Attributes | myBall | Operations | main | BallWorld | paint | pause |
As can be seen above, the BallWorld class will be implemented to have a single variable, an object of the Ball class. It will implement each operation as a method. 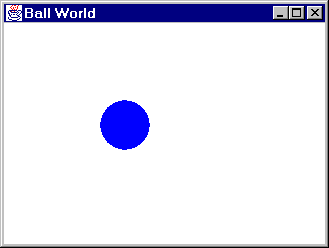
When run version 1 of our BallWorld will simply show a graphical representation of a Ball object in a window for a few seconds, and then the application will terminate. The screen when the application runs should look as follows: The "main" method required for Java applications Every Java application requires one class to have a "main" method -- it is by following the instructions in the "main" method that the Java run-time interpreter knows which instruction to execute first. As we shall investigate in a later unit, Java applets determine their first instruction in a different way. In a nutshell, for version1 of our BallWorld application we wish to do the following: create a window of a certain size show that window on screen create an instance of a Ball class display a representation of the current state (i.e. position, size and colour) of the ball object in our window pause for a short while (so we can see what the application has done) terminate the application and close the window
Although the above list of actions has been written in a simple sequence, due to the nature of object-oriented programming, and its implementation in the Java programming language, some of these actions will be performed in one method, and others in another and so on. Each method does the following: the "main" method creates a new BallWorld object and causes this object's window to be shown on screen (as opposed to the window being hidden) the "BallWorld" method is the constructor method for the class BallWorld, i.e. it does all the things necessary to set up (initialise) the application For our version 1 of BallWorld, the method "BallWorld" defines the dimensions of its window and also creates an instance of a Ball object the "paint" method displays a representation of the BallWorld application on the given graphics object (area of the window). For our version 1 of BallWorld, the only thing we need to paint is our instance of a Ball object the "pause" method causes the application to pause for a given number of milliseconds -- this method allows you to slow down or speed up the application (since each person's PC is set up in a different way, and will be faster or slower according to such features and processor speed and memory)
Implementation of BallWorld in Java - version 1 The Java source file "BallWorld.java" (version 1) looks as follows, although for now we have replaced the statements of each method with a comment. The full source file for "BallWorld.java - version 1" is available for viewing (and can be opened in the Kawa editor). // BallWorld.java public class BallWorld extends Frame { /////////////////// variables /////////// Ball myBall; ///////////////////// methods ////////// void main (String [] args) { // create and display window for a new application object } BallWorld () { // set up window and create a new //instance of class Ball } void paint(Graphics g) { // display things on window, pause, //then terminate program } void pause(int numMilliseconds) { // pause for a given amount of time
}
} // end of class definition
We shall not go into all the details of the implementation of the BallWorld methods (although by the end of the module you will be able to understand all aspects of this application). For now we shall look at particular statements of the BallWorld methods, to illustrate object concepts in Java, and the process of incrementally refining a Java application. A closer look at the methods of "BallWorld.java" Some fundamental object concepts are: An of course, the object concept of the operation is implemented as a method in Java. Each of these features is illustrated in the implementation of the BallWorld class. The "BallWorld" method is implemented as: ////////// method SimpleBall /////////// BallWorld () { // set size and title of our application window setSize(600, 400); setTitle("Ball World"); // make "myBall" a reference to a new instance of ball myBall = new Ball(100, 100, 50, 4, 5); } The second to last line shows the variable "myBall" referring to a new instance of the "Ball" class -- being set up with centre (100,100), radius 50 and movement of (4, 5). This line: myBall = new Ball(100, 100, 50, 4, 5); shows how to create an object in Java -- i.e. by the message "new <Classname>", in this case "new Ball" passed with arguments to set up a new Ball object. 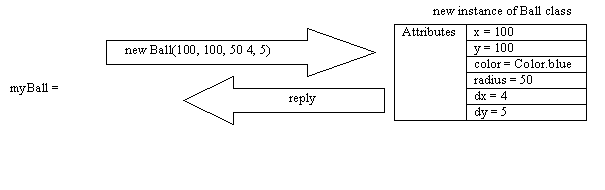 The method (i.e. the Ball method "Ball") returns a reply that is a reference to where this new object has been created (some place in the computer's memory). The variable "myBall" is given this reference, so "myBall" now refers to an instance of the Ball class. The identifier of our variable, "myBall" is the way we can refer to this individual object. The concept of individuality, and its implementation in the identifiers of Java variables is important, since potentially we could have 2 instances of the Ball class, whose attributes are the same, same centre coordinates, same radius, same colour, same movement values, but they are still two different balls. If one were referred to by a variable "ball1" and the other "ball2", we can still individually refer to each instance of the class Ball.
By the way, the "setSize" and "setTitle" statements in the "BallWorld" method simply determine the size of the applications window (600 by 400 pixels), and the title of the window ("Ball World"). Back to top 
RITSEC - Global Campus Copyright ?1999 RITSEC- Middlesex University. All rights reserved. webmaster@globalcampus.com.eg |