The "BallWorld" class - version 7 Since we have made working with 2 Ball objects easy, we shall now generalise our application to work with 5 balls. So when run our application will look something like the following: 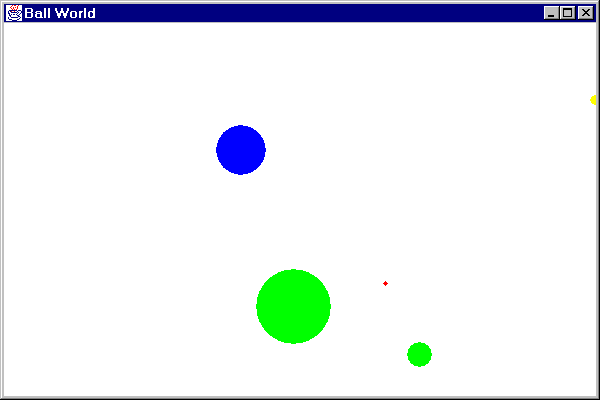
Rather than create a separate variable for each of 5 balls (since eventually we may wish to create an application with 100s or 1000s of balls!), we shall use something called an "array". The details of arrays are delt with in a later unit -- for now just understand that they can stored references to as many objects as we wish. So we need to declare our array variable to stored references to instances of the Ball class: private Ball balls[]; In out BallWorld constructor, we need to state the size of our array: balls = new Ball[5]; We also need to make our array refer to five new Ball objects (we'll make them different sizes, start at different positions, and move in different ways): balls[0] = new Ball(100, 100, 50, 4, 5); balls[1] = new Ball(200, 200, 25, -3, -2); balls[2] = new Ball(50, 50, 5, 1, -1); balls[3] = new Ball(200, 50, 10, -10, -15); balls[4] = new Ball(75, 200, 75, -1, 1); To distinguish them, we can make some of them different colours (we'll leave the first instance, "balls[0]" as the default blue colour): balls[1].setColor( Color.green ); balls[2].setColor( Color.red ); balls[3].setColor( Color.yellow ); balls[4].setColor( Color.green ); Now all we need to do is amend our "paint" method to paint, move and bounce each of the objects referred to by the "balls" array. The "paint" method now looks as follows: public void paint(Graphics g) { // NEW NEW NEW - display and move all 5 balls for( int i = 0; i < balls.length; i++) { balls[i].paint( g ); balls[i].move(); } pause( 20 ); // NEW NEW NEW - call method "bounce" for all 5 balls for( int i = 0; i < balls.length; i++) bounce( balls[i] ); counter = counter + 1; if (counter < 500) repaint(); else System.exit(0); } Note that we are using a "loop" statement (which are introduce in a later unit) to send the "paint" and "move" messages to each ball. Likewise, we are using a loop statement to call method "bounce" with each object the "balls" array refers to. The full source file for "BallWorld.java - version 7" is available for viewing (and can be opened in the Kawa editor). BallWorld version 7: Activity
Back to top 
RITSEC - Global Campus Copyright ?1999 RITSEC- Middlesex University. All rights reserved. webmaster@globalcampus.com.eg |